How to Read a String From a User Input Variable and Store It in a Seperate Variable C++
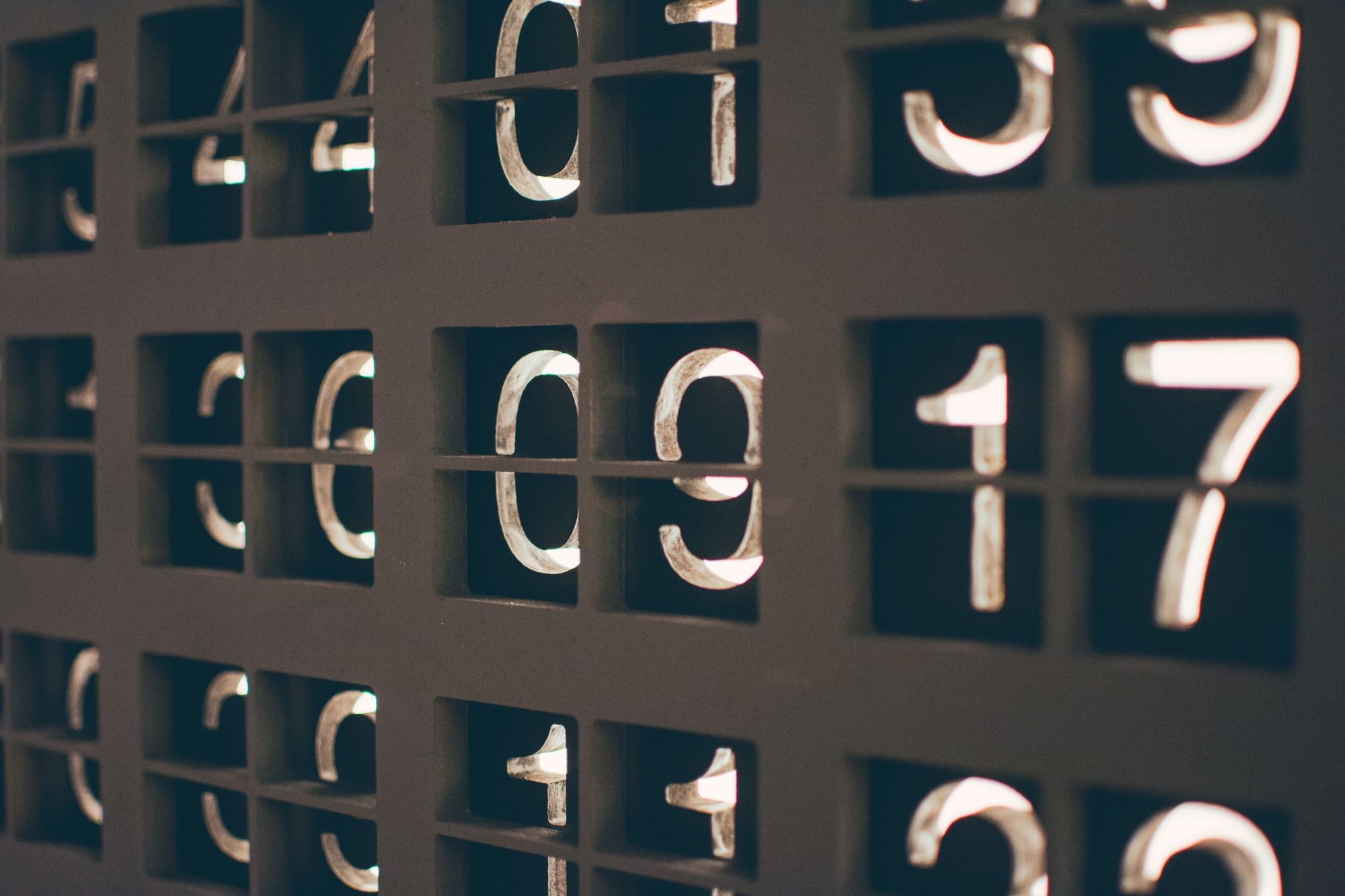
When y'all're coding in C++, there will often exist times when you'll desire to convert one data blazon to a different ane.
In this article yous'll learn how to convert a string to an integer in C++ by seeing 2 of the almost popular ways to practise so.
Let's get started!
Information types in C++
The C++ programming language has a few congenital-in information types:
-
int
, for integer (whole) numbers (for example 10, 150) -
double
, for floating point numbers (for example 5.0, 4.5) -
char
, for single characters (for case 'D', '!') -
string
, for a sequence of characters (for example "Hello") -
bool
, for boolean values (truthful or false)
C++ is a strongly typed programming language, meaning that when you create a variable you take to explicitely declare what type of value will be stored in it.
How to declare and initialize int
southward in C++
To declare an int
variable in C++ you need to offset write the information type of the variable – int
in this instance. This will let the compiler know what kind of values the variable can store and therefore what actions it can take.
Side by side, you demand give the variable a proper name.
Lastly, don't forget the semicolon to end the statement!
#include <iostream> int chief() { int age; }
You lot tin can then give the variable you created a value, like so:
#include <iostream> int main() { int age; historic period = 28; }
Instead of doing these actions as carve up steps, you can combine them by initializing the variable and finally press the issue:
// a header file that enables the employ of functions for outputing information //east.g. cout or inputing information eastward.k. cin #include <iostream> // a namespace argument; you won't take to use the std:: prefix using namespace std; int principal() { // start of main function of the program int historic period = 28; // initialize a variable. //Initializing is providing the type,name and value of the varibale in one go. // output to the console: "My age is 28",using chaining, << cout << "My historic period is: " << age << endl; }// end the primary part
How to declare and initialize string
s in C++
Strings are a drove of individual characters.
Declaring strings in C++ works very similarly to declaring and initializing int
s, which you saw in the section to a higher place.
The C++ standard library provides a cord
class. In order to employ the string data type, y'all'll have to include the <cord>
header library at the top of your file, later #include <iostream>
.
After including that header file, you lot can too add using namespace std;
which y'all saw before.
Among other things, after adding this line, you won't have to utilise std::string
when creating a string variable – just string
solitary.
#include <iostream> #include <string> using namespace std; int main() { //declare a string variable string greeting; greeting = "Hello"; //the `=` is the consignment operator,assigning the value to the variable }
Or you tin initialize a cord variable and impress it to the console:
#include <iostream> #include <string> using namespace std; int principal() { //initialize a string variable cord greeting = "Hullo"; //output "Hello" to the console cout << greeting << endl; }
How to catechumen a string to an integer
As mentioned before, C++ is a strongly typed language.
If you endeavour to give a value that doesn't align with the data blazon, you'll become an error.
Also, converting a string to an integer is not as uncomplicated as using type casting, which you tin can use when converting double
southward to int
south.
For example, you cannot practise this:
#include <iostream> #include <string> using namespace std; int main() { cord str = "7"; int num; num = (int) str; }
The fault after compiling will be:
hellp.cpp:9:ten: fault: no matching conversion for C-style bandage from 'std::__1::string' (aka 'basic_string<char, char_traits<char>, allocator<char> >') to 'int' num = (int) str; ^~~~~~~~~ /Library/Developer/CommandLineTools/usr/bin/../include/c++/v1/string:875:5: note: candidate function operator __self_view() const _NOEXCEPT { return __self_view(data(), size()); } ^ 1 error generated.
There are a few ways to catechumen a cord to an int, and y'all'll run across two of them mentioned in the sections that follow.
How to catechumen a string to an int using the stoi()
function
One constructive style to convert a string object into a numeral int is to apply the stoi()
function.
This method is commonly used for newer versions of C++, with is existence introduced with C++11.
It takes as input a string value and returns as output the integer version of it.
#include <iostream> #include <cord> using namespace std; int primary() { // a string variable named str string str = "seven"; //print to the console cout << "I am a string " << str << endl; //convert the string str variable to have an int value //place the new value in a new variable that holds int values, named num int num = stoi(str); //print to the console cout << "I am an int " << num << endl; }
Output:
I am a string seven I am an int 7
How to convert a string to an int using the stringstream
class
The stringstream
grade is more often than not used in earlier versions of C++. It works by performing inputs and outputs on strings.
To use it, you lot first have to include the sstream
library at the meridian of your program by adding the line #include <sstream>
.
You so add the stringstream
and create an stringstream
object, which will hold the value of the string you want to catechumen to an int and will be used during the process of converting it to an int.
You lot use the <<
operator to extract the cord from the cord variable.
Lastly, you use the >>
operator to input the newly converted int value to the int variable.
#include <iostream> #include <string> #include <sstream> // this volition allow you to use stringstream in your program using namespace std; int main() { //create a stringstream object, to input/output strings stringstream ss; // a variable named str, that is of string information type cord str = "7"; // a variable named num, that is of int data type int num; //extract the cord from the str variable (input the string in the stream) ss << str; // place the converted value to the int variable ss >> num; //print to the consloe cout << num << endl; // prints the intiger value vii }
Conclusion
And there yous have it! You lot have seen two simple ways to convert a string to an integer in C++.
If you're looking to learn more about the C++ programming language, cheque out this 4-hour grade on freeCodeCamp's YouTube channel.
Thank you for reading and happy learning 😊
Learn to lawmaking for costless. freeCodeCamp'due south open up source curriculum has helped more 40,000 people get jobs every bit developers. Become started
How to Read a String From a User Input Variable and Store It in a Seperate Variable C++
Source: https://www.freecodecamp.org/news/string-to-int-in-c-how-to-convert-a-string-to-an-integer-example/